Console: Expanding Logging
I deployed a small test method and wanted to know some debug information in production of how long certain loading and processing actions were taking and could have used the old standby of logging to the browser with the use of console.log
, but now there are many more and abilities than first glance and opens up different use cases.
const fn = (msg) => {
console.debug(`${msg ?? ""} debug level message only`);
};
const fnError = (msg) => {
console.error(`${msg ?? ""} error level message only`);
};
const fnWarn = (msg) => {
console.warn(`${msg ?? ""} warn level message only`);
};
const fnLog = (msg) => {
console.log(`${msg ?? ""} info level message only`);
};
const fnTrace = (msg) => {
console.trace(`${msg ?? ""} info level message with context`);
};
const trackDelay = (time) => {
console.time();
setTimeout(() => {
console.timeEnd();
}, time);
};
const trackSteps = (time) => {
const timerName = "tracker";
console.time(timerName);
setTimeout(() => {
console.timeLog(timerName);
}, time);
console.timeLog(timerName);
};
Produces no visible output at normal opening of the console
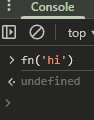
However using the expanded console options you can see the message was created as expected
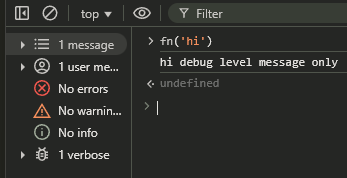
Now using the and error
level message is higher and will display as in this case
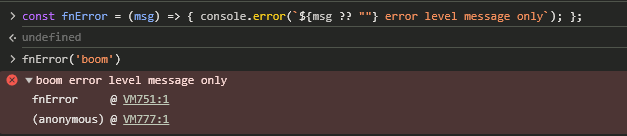
At warn
level

info
and trace
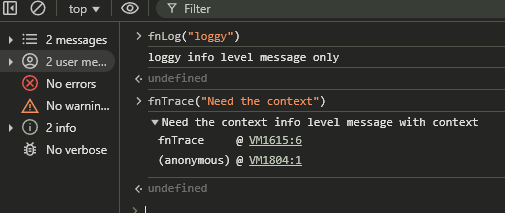
Timing execution time time()
and timeEnd()
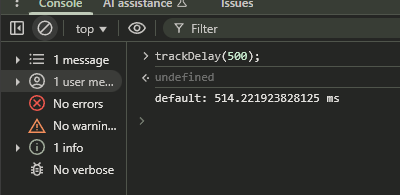
Track multiple timings with a unique timer using time()
and timeLog()
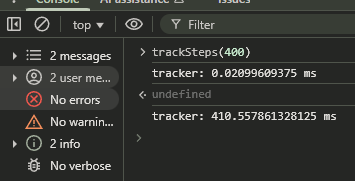